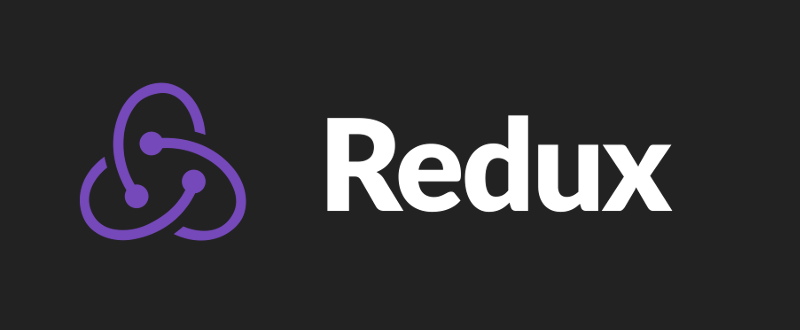
Redux is a popular state management library often used with JavaScript frameworks like React, Angular, and Vue.js. It provides a predictable way to manage the state of your application, making it easier to understand, debug, and maintain.
What is Redux?
Redux is a predictable state container for JavaScript applications. It helps you manage the state of your application in a single, centralized store. This is particularly useful for complex applications where state needs to be shared across multiple components.
The core principles of Redux are:
- Single Source of Truth: The state of your entire application is stored in a single object tree within a single store.
- State is Read-Only: The only way to change the state is to emit an action, an object describing what happened.
- Changes are Made with Pure Functions: To specify how the state tree is transformed by actions, you write pure reducers.
Why Use Redux?
Redux is not always necessary, especially for smaller applications. However, it becomes extremely valuable in scenarios where:
- The application state becomes difficult to manage with just React’s built-in state management.
- You need to maintain a consistent state across a large and complex application.
- You want to implement a time-travel debugging feature (e.g., going back and forth through actions to see how the state changes).
How Does Redux Work?
Redux works by having a single store where the state of the entire application is kept. Components can access the state and dispatch actions to modify it. Here's a simple breakdown of how Redux works:
- Store: The store holds the state of the application.
- Actions: Actions are payloads of information that send data from your application to the store.
- Reducers: Reducers specify how the application's state changes in response to actions.
- Dispatch: Components dispatch actions to the store to trigger state changes.
- Selectors: Selectors are functions that extract, derive, or manipulate data from the state.
Redux Example: A Simple Counter Application
Let’s walk through a basic example of how Redux can be used to manage state in a simple counter application.
Step 1: Install Redux
First, you need to install Redux and React-Redux (a binding library to connect Redux with React).
npm install redux react-redux
Step 2: Create Actions
Actions are plain JavaScript objects that represent the changes you want to make to the state.
// actions.js
export const increment = () => {
return {
type: 'INCREMENT',
};
};
export const decrement = () => {
return {
type: 'DECREMENT',
};
};
Step 3: Create a Reducer
Reducers are functions that determine how the state changes in response to an action.
// reducer.js
const counterReducer = (state = 0, action) => {
switch (action.type) {
case 'INCREMENT':
return state + 1;
case 'DECREMENT':
return state - 1;
default:
return state;
}
};
export default counterReducer;
Step 4: Create the Store
The store is created using the reducer. You can also apply middleware here if needed.
// store.js
import { createStore } from 'redux';
import counterReducer from './reducer';
const store = createStore(counterReducer);
export default store;
Step 5: Connecting Redux with React
Using react-redux
, you can connect your React components with the Redux store.
// Counter.js
import React from 'react';
import { useSelector, useDispatch } from 'react-redux';
import { increment, decrement } from './actions';
function Counter() {
const count = useSelector((state) => state);
const dispatch = useDispatch();
return (
<div>
<h1>{count}</h1>
<button onClick={() => dispatch(increment())}>Increment</button>
<button onClick={() => dispatch(decrement())}>Decrement</button>
</div>
);
}
export default Counter;
Step 6: Wrap the Application with the Provider
Finally, wrap your application with the Provider
component from react-redux
to give it access to the store.
// App.js
import React from 'react';
import { Provider } from 'react-redux';
import store from './store';
import Counter from './Counter';
function App() {
return (
<Provider store={store}>
<Counter />
</Provider>
);
}
export default App;
Conclusion
Redux provides a robust framework for managing state in JavaScript applications, particularly when your application grows in complexity. While it introduces some boilerplate code, the benefits of predictability, maintainability, and easier debugging often outweigh the initial setup costs.