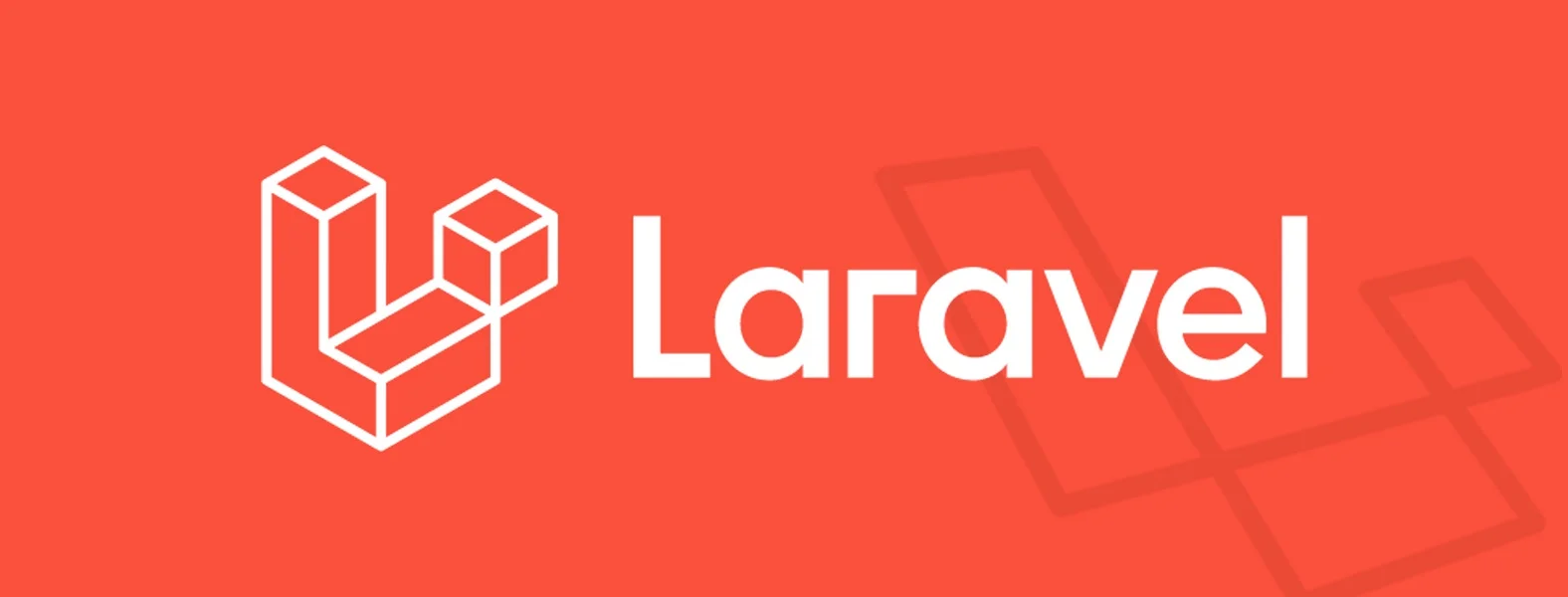
Laravel, a popular PHP framework, is known for its elegant syntax and robust features, making it an excellent choice for building RESTful APIs. One of the key aspects of developing APIs is maintaining a standard response format to ensure consistency, ease of integration, and a better developer experience. In this article, we'll explore how to achieve and maintain a standard REST API response format in Laravel.
Importance of a Standard Response Format
Maintaining a standard response format in your REST API is crucial for several reasons:
- Consistency: Uniform responses across different endpoints make it easier for developers to consume your API.
- Error Handling: Standardizing error responses helps in better debugging and enhances the overall user experience.
- Documentation and Integration: Consistent responses simplify the documentation process and make it easier for third-party developers to integrate with your API.
- Scalability: As your API grows, maintaining a standard format ensures new endpoints adhere to the same principles, reducing technical debt and simplifying maintenance.
Setting Up a Standard Response Format in Laravel
Here are the steps to set up and maintain a standard response format in your Laravel application:
1. Define a Response Structure
Start by defining the structure of your API responses. A typical response might include:
status
: Indicates the success or failure of the request.data
: Contains the main content of the response.error
: Details of any errors encountered.metadata
: Additional information such as pagination details, request IDs, and timestamps.links
: Hyperlinks to related resources.
2. Create a Response Helper
To ensure consistent responses, create a helper function or a trait that formats the responses according to your predefined structure. You can place this in a service provider or a dedicated helper file.
<?php
namespace App\Helpers;
class ApiResponse
{
public static function success($data, $metadata = [], $links = [])
{
return response()->json([
'status' => 'success',
'data' => $data,
'metadata' => $metadata,
'links' => $links
], 200);
}
public static function error($message, $code = 400, $details = [])
{
return response()->json([
'status' => 'error',
'error' => [
'code' => $code,
'message' => $message,
'details' => $details
],
'metadata' => [
'timestamp' => now(),
]
], $code);
}
}
3. Use the Response Helper in Controllers
Utilize the response helper in your controllers to maintain consistency. Here's an example of how to use it in a typical controller action:
<?php
namespace App\Http\Controllers;
use App\Helpers\ApiResponse;
use App\Models\Item;
use Illuminate\Http\Request;
class ItemController extends Controller
{
public function index()
{
$items = Item::all();
return ApiResponse::success($items, ['count' => $items->count()]);
}
public function show($id)
{
$item = Item::find($id);
if (!$item) {
return ApiResponse::error('Resource not found', 404);
}
return ApiResponse::success($item);
}
public function store(Request $request)
{
$validatedData = $request->validate([
'name' => 'required|string|max:255',
'description' => 'required|string|max:1000',
]);
$item = Item::create($validatedData);
return ApiResponse::success($item, [], [
'self' => route('items.show', $item->id)
]);
}
}
4. Handle Exceptions
To ensure that exceptions are handled consistently, you can customize the exception handler in app/Exceptions/Handler.php
.
<?php
namespace App\Exceptions;
use App\Helpers\ApiResponse;
use Illuminate\Foundation\Exceptions\Handler as ExceptionHandler;
use Throwable;
class Handler extends ExceptionHandler
{
public function render($request, Throwable $exception)
{
$code = $exception->getCode() ?: 500;
$message = $exception->getMessage() ?: 'Internal Server Error';
return ApiResponse::error($message, $code);
}
}
5. Document the API
Documenting your API is crucial for helping developers understand how to interact with it. Tools like Swagger can be used to generate interactive API documentation that follows the standard response format.
openapi: 3.0.0
info:
title: Sample API
version: 1.0.0
paths:
/items:
get:
summary: Get all items
responses:
'200':
description: A list of items
content:
application/json:
schema:
type: object
properties:
status:
type: string
data:
type: array
items:
$ref: '#/components/schemas/Item'
metadata:
type: object
links:
type: object
components:
schemas:
Item:
type: object
properties:
id:
type: integer
name:
type: string
description:
type: string
Conclusion
Maintaining a standard REST API response format in Laravel is crucial for creating a consistent, predictable, and user-friendly API. By defining a clear response structure, creating helper functions, and ensuring consistent usage across your application, you can enhance the developer experience and simplify the integration process. Additionally, handling exceptions and thoroughly documenting your API further contribute to a robust and maintainable API ecosystem. By following these best practices, you can build and maintain high-quality APIs that meet the needs of your users and developers.