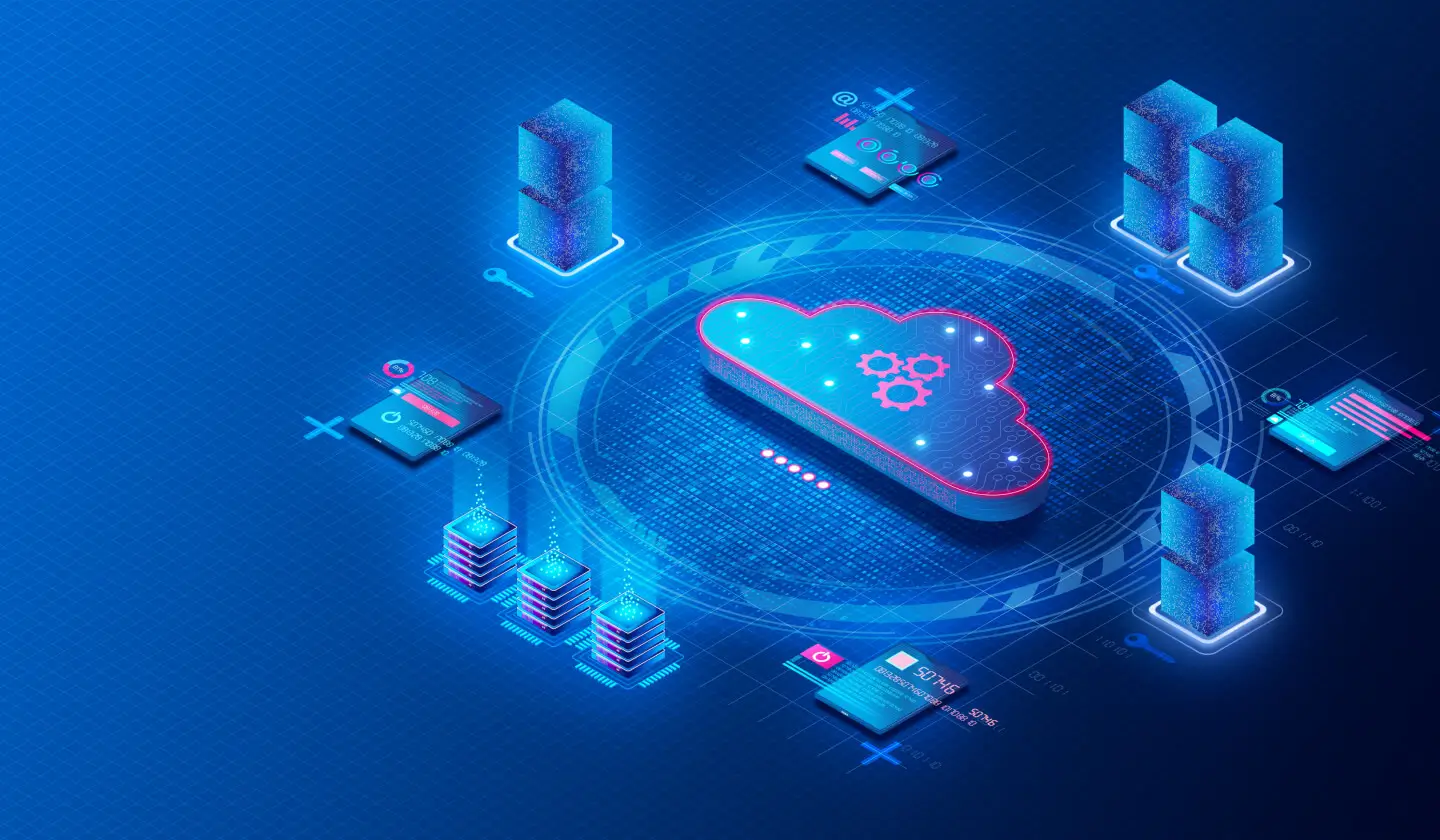
In today's digital age, handling transactions efficiently and securely is paramount for businesses. With AWS's powerful serverless offerings, we can create scalable and robust systems with ease. This article delves into a practical implementation of a receipt generation system using AWS serverless architecture.
Overview
Our architecture is designed to handle transaction data from a payment gateway and generate receipts seamlessly. The key components of this system include:
- API Gateway
- AWS Lambda
- Amazon SQS
- DynamoDB
- Amazon S3
Here's a step-by-step guide to understanding and implementing this architecture.
Architecture Breakdown
The architecture consists of the following components:
- API Gateway: Acts as an entry point for the webhook call from the payment gateway.
- Lambda 1: Processes the initial transaction data and stores it in DynamoDB.
- SQS 1: Manages the queue for processing transactions, ensuring decoupling and reliability.
- Lambda 2: Further processes the transaction data, prepares the receipt, and stores it in Amazon S3.
- Lambda 3: Sends notifications via email, WhatsApp, or SMS.
- Amazon S3: Stores the generated receipts.
- DynamoDB: Stores transaction records and metadata.
Step-by-Step Implementation
-
API Gateway Setup:
- Create an API Gateway to receive webhook calls from the payment gateway.
- Configure the API Gateway to trigger
Lambda 1
upon receiving a POST request.
-
Lambda 1:
- Trigger: API Gateway
- Function: This Lambda function processes the incoming transaction data and stores relevant details in DynamoDB.
import json
import boto3
from botocore.exceptions import ClientError
def lambda_handler(event, context):
dynamodb = boto3.resource('dynamodb')
table = dynamodb.Table('Transactions')
try:
# Parse the transaction data
transaction = json.loads(event['body'])
# Store the transaction data in DynamoDB
table.put_item(Item=transaction)
# Send the transaction data to SQS
sqs = boto3.client('sqs')
response = sqs.send_message(
QueueUrl='https://sqs.region.amazonaws.com/account-id/queue-name',
MessageBody=json.dumps(transaction)
)
return {
'statusCode': 200,
'body': json.dumps('Transaction processed successfully')
}
except ClientError as e:
return {
'statusCode': 500,
'body': json.dumps(f'Error processing transaction: {e}')
}
SQS 1:
- Acts as a buffer between
Lambda 1
andLambda 2
, ensuring reliable processing.
Lambda 2:
- Trigger: SQS 1
- Function: This function retrieves messages from SQS, processes the transaction data, and generates a receipt.
import json
import boto3
import uuid
def lambda_handler(event, context):
s3 = boto3.client('s3')
bucket_name = 'receipt-bucket'
for record in event['Records']:
# Process each transaction
transaction = json.loads(record['body'])
receipt_id = str(uuid.uuid4())
receipt_content = f"Receipt for Transaction ID: {transaction['transaction_id']}\nAmount: {transaction['amount']}\nDate: {transaction['date']}"
# Save the receipt to S3
s3.put_object(Bucket=bucket_name, Key=f'receipts/{receipt_id}.txt', Body=receipt_content)
# Optionally, save metadata to DynamoDB
dynamodb = boto3.resource('dynamodb')
table = dynamodb.Table('Receipts')
table.put_item(Item={'receipt_id': receipt_id, 'transaction_id': transaction['transaction_id'], 's3_key': f'receipts/{receipt_id}.txt'})
Lambda 3:
- Trigger: S3 (Event notification on object creation)
- Function: Sends notifications to users via email, WhatsApp, or SMS.
import json
import boto3
def lambda_handler(event, context):
sns = boto3.client('sns')
for record in event['Records']:
# Get the S3 bucket and object key from the event
bucket = record['s3']['bucket']['name']
key = record['s3']['object']['key']
# Construct the notification message
message = f"Your receipt has been generated and is available at: s3://{bucket}/{key}"
# Send the notification
response = sns.publish(
PhoneNumber='+1234567890', # replace with the actual phone number
Message=message
)
print(f"Message sent with response: {response}")
Benefits of Serverless Architecture
- Scalability: Automatically scales based on demand.
- Cost-Effective: Pay only for what you use.
- Resilience: Managed services with built-in redundancy.
- Reduced Operational Overhead: Focus on business logic rather than server management.
Conclusion
Using AWS serverless services like Lambda, API Gateway, SQS, DynamoDB, and S3, you can build a robust receipt generation system that scales with your business needs. This architecture ensures reliable transaction processing, receipt generation, and user notifications, providing a seamless experience for both the business and its customers.