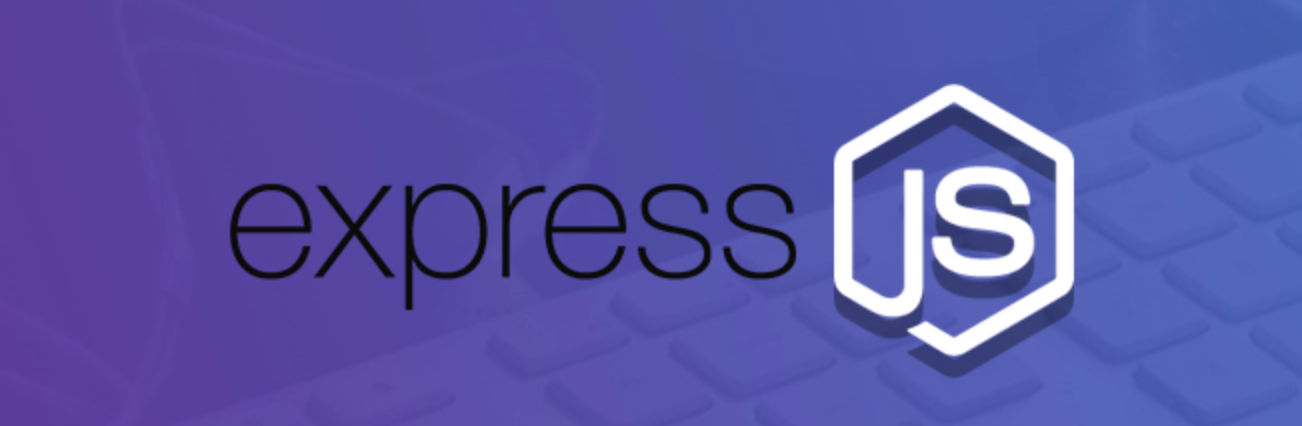
Express.js is a popular web application framework for Node.js, known for its simplicity and flexibility. When building RESTful APIs with Express.js, it's essential to maintain a standard response format. This consistency aids in integration, debugging, and overall user experience. In this article, we'll explore the importance of a standardized response format and how to implement and maintain it in an Express.js application.
Importance of a Standard Response Format
Maintaining a standard response format in your REST API is crucial for several reasons:
- Consistency: Uniform responses across different endpoints make it easier for developers to consume your API.
- Error Handling: Standardized error responses help in better debugging and enhance the overall user experience.
- Documentation and Integration: Consistent responses simplify the documentation process and make it easier for third-party developers to integrate with your API.
- Scalability: As your API grows, maintaining a standard format ensures new endpoints adhere to the same principles, reducing technical debt and simplifying maintenance.
Setting Up a Standard Response Format in Express.js
Here are the steps to set up and maintain a standard response format in your Express.js application:
1. Define a Response Structure
Start by defining the structure of your API responses. A typical response might include:
status
: Indicates the success or failure of the request.data
: Contains the main content of the response.error
: Details of any errors encountered.metadata
: Additional information such as pagination details, request IDs, and timestamps.links
: Hyperlinks to related resources.
2. Create a Response Helper
To ensure consistent responses, create a middleware function that formats the responses according to your predefined structure. This function can be placed in a dedicated middleware directory.
// middleware/responseFormatter.js
const responseFormatter = (req, res, next) => {
res.success = (data, metadata = {}, links = {}) => {
res.status(200).json({
status: 'success',
data,
metadata,
links
});
};
res.error = (message, code = 400, details = {}) => {
res.status(code).json({
status: 'error',
error: {
code,
message,
details
},
metadata: {
timestamp: new Date().toISOString(),
}
});
};
next();
};
module.exports = responseFormatter;
3. Use the Response Helper in Your Application
Apply the response helper middleware globally in your Express.js application to ensure all routes have access to the standard response format.
// app.js
const express = require('express');
const responseFormatter = require('./middleware/responseFormatter');
const app = express();
app.use(express.json());
app.use(responseFormatter);
// Define your routes here
app.listen(3000, () => {
console.log('Server is running on port 3000');
});
4. Implement the Standard Response in Controllers
Use the response helper methods in your route handlers to maintain consistency. Here's an example of how to use it in typical route handlers:
// routes/itemRoutes.js
const express = require('express');
const router = express.Router();
const Item = require('../models/item');
// GET all items
router.get('/', async (req, res) => {
try {
const items = await Item.find();
res.success(items, { count: items.length });
} catch (err) {
res.error('Failed to fetch items', 500, err.message);
}
});
// GET item by ID
router.get('/:id', async (req, res) => {
try {
const item = await Item.findById(req.params.id);
if (!item) {
return res.error('Resource not found', 404);
}
res.success(item);
} catch (err) {
res.error('Failed to fetch item', 500, err.message);
}
});
// POST create a new item
router.post('/', async (req, res) => {
try {
const item = new Item(req.body);
await item.save();
res.success(item, {}, { self: `/api/items/${item._id}` });
} catch (err) {
res.error('Failed to create item', 400, err.message);
}
});
module.exports = router;
5. Handle Exceptions
To ensure that exceptions are handled consistently, create an error-handling middleware in your application.
// middleware/errorHandler.js
const errorHandler = (err, req, res, next) => {
const statusCode = err.statusCode || 500;
const message = err.message || 'Internal Server Error';
res.error(message, statusCode, {
stack: process.env.NODE_ENV === 'production' ? null : err.stack,
});
};
module.exports = errorHandler;
Apply the error-handling middleware in your application.
// app.js
const errorHandler = require('./middleware/errorHandler');
// Other middleware and routes
app.use(errorHandler);
Conclusion
Maintaining a standard REST API response format in Express.js is crucial for creating a consistent, predictable, and user-friendly API. By defining a clear response structure, creating middleware for response formatting, and ensuring consistent usage across your application, you can enhance the developer experience and simplify the integration process. Additionally, handling exceptions and thoroughly documenting your API further contribute to a robust and maintainable API ecosystem. By following these best practices, you can build and maintain high-quality APIs that meet the needs of your users and developers.