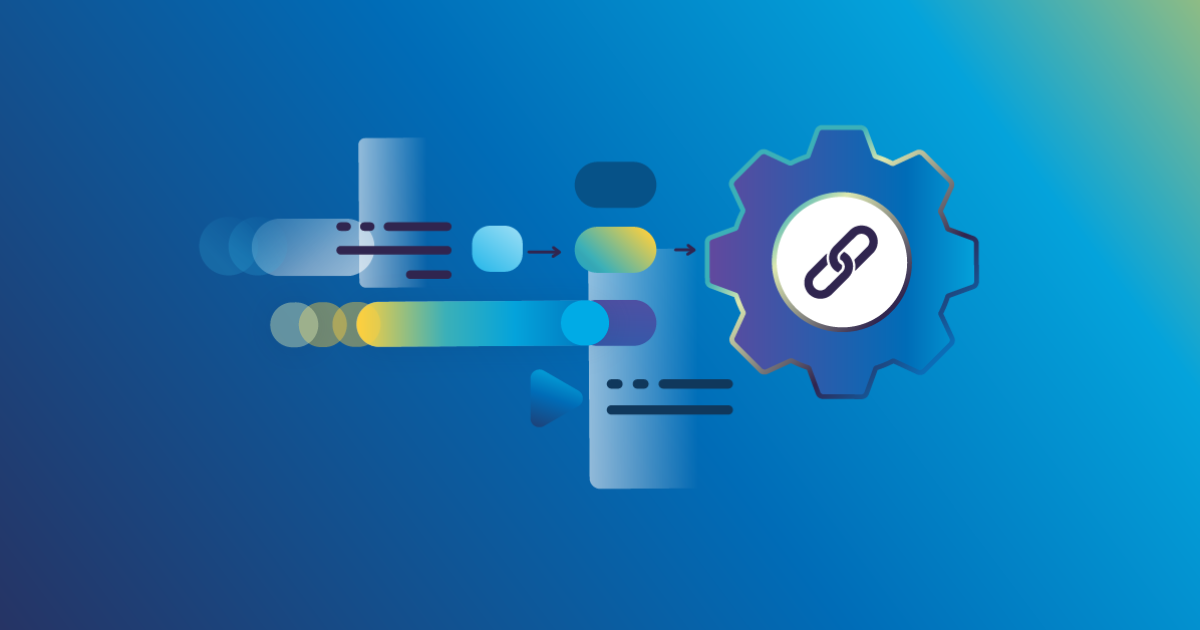
Introduction
LangChain is a powerful framework for working with language models and enables developers to build applications that harness the power of large language models (LLMs) in a structured and customizable way. If you’re working with a folder of documents, loading them efficiently into LangChain can be crucial, especially for tasks like search, summarization, question-answering, or knowledge extraction. This article will guide you through the steps to load a folder of documents into LangChain, ensuring you can manage and process large datasets seamlessly.
Prerequisites
-
Python 3.8+ installed.
-
LangChain Library: Make sure LangChain is installed in your environment. You can install it with:
pip install langchain
-
Document Types: Ensure your folder contains document types compatible with LangChain (e.g.,
.txt
,.pdf
,.docx
,.csv
, etc.).
Step 1: Setting Up Document Loaders
LangChain provides several document loaders to handle different file formats. First, you need to import the appropriate document loader for the type of files in your folder. If you have multiple file types, you may need to use multiple loaders.
Example: Loading .txt
and .pdf
Files
For example, if your folder has .txt
and .pdf
files, use TextLoader
and PyMuPDFLoader
(for .pdf
), respectively.
from langchain.document_loaders import TextLoader, PyMuPDFLoader
Step 2: Configuring the Directory Loader
LangChain’s DirectoryLoader
makes it easy to load all files from a specific directory by specifying loaders for different file types.
Setting up DirectoryLoader with Multiple File Types
You can set up DirectoryLoader
to load specific file types by using glob
patterns.
from langchain.document_loaders import DirectoryLoader
# Define a directory path
directory_path = "./documents"
# Create the DirectoryLoader, specifying loaders for each file type
loader = DirectoryLoader(
directory_path,
glob="**/*", # This pattern loads all files; modify as needed
loader_mapping={
".txt": TextLoader, # For .txt files
".pdf": PyMuPDFLoader # For .pdf files
}
)
In this setup:
directory_path
is the path to the folder containing your documents.glob
is a pattern matching all files. You can customize it (e.g.,*.pdf
,*.txt
) if needed.
Step 3: Loading Documents into LangChain
Now that the DirectoryLoader
is configured, you can load all documents using the load()
method. This method will go through each file, apply the appropriate loader, and load the documents into LangChain as a list of documents.
# Load documents
documents = loader.load()
print(f"Loaded {len(documents)} documents.")
Each document is represented as a dictionary containing metadata and the content of the document. Now, documents
can be fed into different components within LangChain for further processing.
Step 4: Processing Loaded Documents
Once your documents are loaded, you can start leveraging LangChain’s capabilities for various NLP tasks. Here’s a simple example of printing document content and metadata:
for doc in documents:
print("Content:", doc["content"][:100]) # Print the first 100 characters of content
print("Metadata:", doc["metadata"])
print()
Step 5: Advanced Usage and Custom Loaders
LangChain supports custom loaders for special file types. To create a custom loader:
- Subclass
BaseLoader
. - Implement the
load()
method to read your file type.
from langchain.document_loaders import BaseLoader
class CustomLoader(BaseLoader):
def __init__(self, file_path):
self.file_path = file_path
def load(self):
# Implement custom loading logic
content = "Your custom content loading logic here"
return [{"content": content, "metadata": {"source": self.file_path}}]
Use this custom loader in your DirectoryLoader
setup if you need it for specialized document types.
Conclusion
Loading a folder of documents into LangChain is straightforward with the DirectoryLoader
. By mapping different file types to their appropriate loaders, you can easily prepare documents for NLP tasks, and the flexibility to create custom loaders further enhances its adaptability.
This setup helps you streamline workflows involving large datasets or diverse document types, setting up your application to leverage LangChain’s capabilities to the fullest.