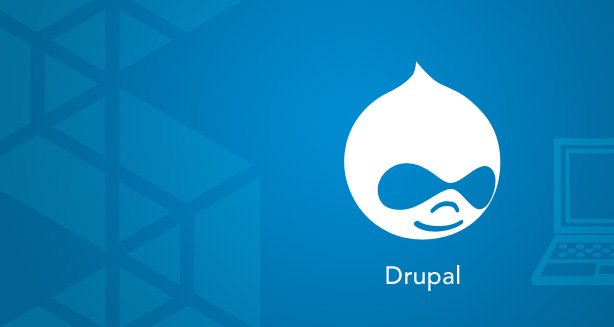
Drupal is a content management system that allows you to create and manage website content in an organized way. One of the ways to create content in Drupal is by creating a node programmatically. A node is a fundamental building block of a Drupal website and represents a piece of content such as an article, a page, or a product.
To create a node programmatically in Drupal 10, you will need to use the Drupal's Entity API, specifically the Node class. Here's an example of how you can create a basic node:
use Drupal\node\Entity\Node;
// Create a new node object.
$node = Node::create([
'type' => 'article',
'title' => 'My programmatically created node',
'body' => [
'value' => 'This is the body of my programmatically created node.',
'format' => 'full_html',
],
]);
// Save the node.
$node->save();
In this example, we are creating a node of type "article" with the title "My programmatically created node" and the body "This is the body of my programmatically created node." The body field has a 'value' and 'format' properties. Value is the content you want to save and format is the text format like full_html or plain_text.
You can also add fields or extra data like images, files, or references to other entities in your node by setting their values in the Node object before calling the save()
method.
use Drupal\file\Entity\File;
// Create a new file object
$file = File::create([
'uri' => 'public://example.jpg',
'status' => 1,
]);
$file->save();
// add file object to the node object
$node->set('field_image', [
'target_id' => $file->id(),
]);
In this example, we create an image field on the node and assign the image to it, here "field_image" is the field machine name you have defined on your content type.
Once you have created and configured your node, you can use the save()
method to persist it to the database.
It's important to note that the code should be executed by a proper script or form submit callback or Drush command and not directly on the template file or web page.
Please note that this is a very basic example, you may want to look into more complex examples or other modules if your needs are more specific.