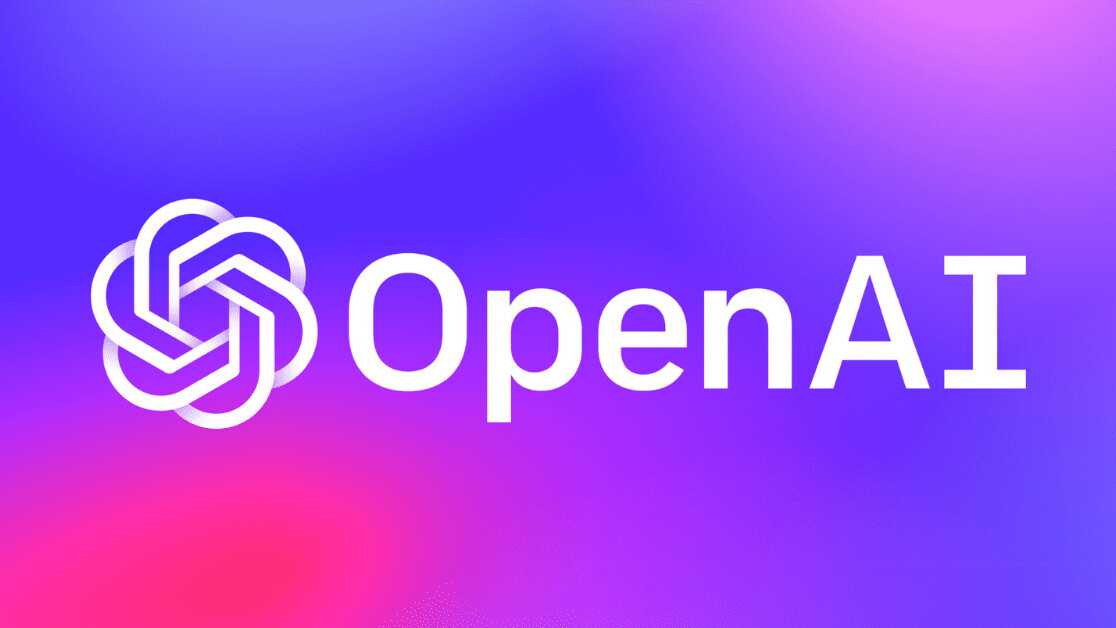
OpenAI's GPT models are among the most advanced AI models available for natural language processing (NLP). While directly training a GPT model from scratch requires significant computational resources, fine-tuning a pre-trained model on your custom data is a more practical and efficient approach. This tutorial will guide you through the process of fine-tuning an OpenAI GPT model using Python and the Hugging Face transformers
library.
Step 1: Set Up the Environment
First, ensure you have the necessary libraries installed. You will need transformers
, datasets
, and torch
for this tutorial. You can install them using pip:
pip install transformers datasets torch
Step 2: Import Necessary Libraries
Begin by importing the necessary libraries:
import torch
from transformers import GPT2Tokenizer, GPT2LMHeadModel, Trainer, TrainingArguments
from datasets import load_dataset, Dataset
Step 3: Prepare Your Custom Dataset
Assume you have a text file named custom_data.txt
with your custom data. Each line in this file represents a separate training example.
# Load your custom data into a list
with open('custom_data.txt', 'r', encoding='utf-8') as f:
custom_data = f.readlines()
# Create a dataset from the custom data
dataset = Dataset.from_dict({"text": custom_data})
Step 4: Load and Tokenize the Data
Next, load the pre-trained GPT-2 tokenizer and model. Tokenize your dataset using the GPT-2 tokenizer.
# Load pre-trained GPT-2 tokenizer
tokenizer = GPT2Tokenizer.from_pretrained('gpt2')
# Tokenize the dataset
def tokenize_function(examples):
return tokenizer(examples['text'], padding='max_length', truncation=True, max_length=512)
tokenized_datasets = dataset.map(tokenize_function, batched=True)
Step 5: Load the Pre-trained GPT-2 Model
Load the pre-trained GPT-2 model. We'll fine-tune this model on the custom dataset.
# Load pre-trained GPT-2 model
model = GPT2LMHeadModel.from_pretrained('gpt2')
Step 6: Set Up Training Arguments
Define the training arguments. These control the fine-tuning process, such as the number of epochs, learning rate, and batch size.
# Define training arguments
training_args = TrainingArguments(
output_dir='./results', # output directory
num_train_epochs=3, # number of training epochs
per_device_train_batch_size=2, # batch size for training
save_steps=10_000, # number of updates steps before checkpoint
save_total_limit=2, # limit the total amount of checkpoints
prediction_loss_only=True,
)
Step 7: Fine-Tune the Model
Create a Trainer
instance and start fine-tuning the model.
# Initialize the Trainer
trainer = Trainer(
model=model,
args=training_args,
train_dataset=tokenized_datasets,
)
# Start training
trainer.train()
Step 8: Evaluate the Model
After fine-tuning, evaluate the model's performance on a sample input.
# Save the fine-tuned model and tokenizer
model.save_pretrained("./fine_tuned_model")
tokenizer.save_pretrained("./fine_tuned_model")
# Load the fine-tuned model and tokenizer for evaluation
fine_tuned_model = GPT2LMHeadModel.from_pretrained("./fine_tuned_model")
fine_tuned_tokenizer = GPT2Tokenizer.from_pretrained("./fine_tuned_model")
# Test the fine-tuned model on a sample input
input_text = "Once upon a time"
input_ids = fine_tuned_tokenizer.encode(input_text, return_tensors='pt')
# Generate text
output = fine_tuned_model.generate(input_ids, max_length=50, num_return_sequences=1)
# Decode the generated text
generated_text = fine_tuned_tokenizer.decode(output[0], skip_special_tokens=True)
print(generated_text)
Conclusion
Fine-tuning an OpenAI GPT model on your custom data can significantly improve its performance on specific tasks or domains. This tutorial demonstrated how to use Python and the Hugging Face transformers
library to fine-tune a GPT-2 model with a custom dataset. By following these steps, you can leverage the power of advanced language models to suit your unique needs and applications.