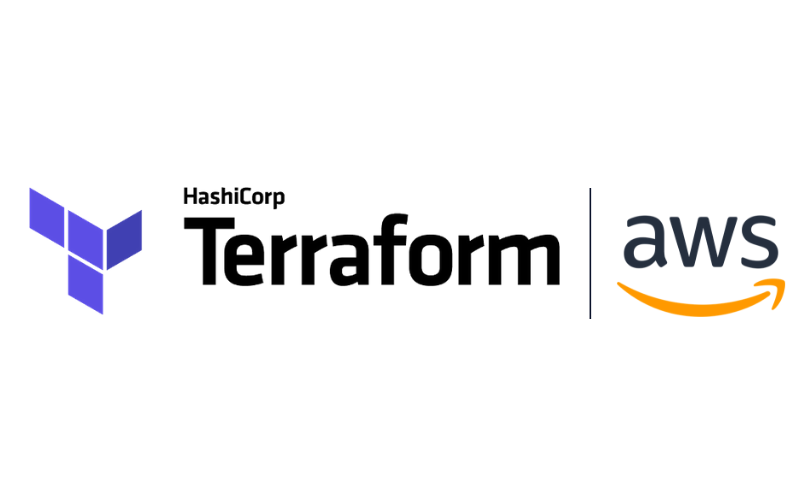
Managing security in the cloud is one of the most important tasks for any organization, and if you’re working with AWS, the job is even more crucial. Terraform, a popular infrastructure-as-code (IaC) tool, can help simplify and automate this task by allowing you to define your AWS infrastructure and security settings in a repeatable, consistent manner. In this guide, we’ll walk through some practical tips on how to use Terraform to enhance the security of your AWS environment.
1. Start with the Principle of Least Privilege
One of the golden rules in security is the Principle of Least Privilege. This means giving users, services, and applications the minimum level of access they need to do their job, and no more. In AWS, this is mostly about properly configuring Identity and Access Management (IAM) roles and policies.
Let’s say you’re setting up an IAM policy to allow read-only access to an S3 bucket. Instead of granting broad permissions, Terraform allows you to craft the exact permissions required.
Example: Defining a Minimal S3 Read-Only Policy
resource "aws_iam_policy" "read_only_s3" {
name = "ReadOnlyS3"
policy = jsonencode({
"Version": "2012-10-17",
"Statement": [
{
"Action": "s3:GetObject",
"Effect": "Allow",
"Resource": "arn:aws:s3:::my-bucket/*"
}
]
})
}
This policy does exactly what it says on the tin: allows read-only access to objects in a specific S3 bucket.
Key Takeaways:
- Always grant the least amount of privilege necessary.
- Regularly review IAM policies to ensure they’re not overly permissive.
2. Handle Secrets Securely
One of the biggest security risks is exposing sensitive information like API keys, database credentials, or encryption keys. Hardcoding these secrets in your Terraform files is a big no-no. Instead, you should use AWS services like Secrets Manager or Systems Manager (SSM) Parameter Store to store and manage secrets.
Example: Using AWS Secrets Manager in Terraform
resource "aws_secretsmanager_secret" "db_password" {
name = "db-password"
}
resource "aws_secretsmanager_secret_version" "db_password_version" {
secret_id = aws_secretsmanager_secret.db_password.id
secret_string = "SuperSecretPassword123"
}
resource "aws_db_instance" "mydb" {
password = aws_secretsmanager_secret_version.db_password_version.secret_string
...
}
Here, Terraform pulls the database password from Secrets Manager instead of hardcoding it, ensuring it stays secure.
Key Takeaways:
- Use Secrets Manager or Parameter Store to manage sensitive information.
- Never hardcode credentials or sensitive data in your configuration files.
3. Encrypt Everything
Encryption is another key part of keeping your data secure. Whether it’s encrypting data at rest (e.g., stored in S3, RDS, or EBS) or data in transit (e.g., through HTTPS), Terraform makes it easy to configure encryption for AWS services.
Example: Enabling S3 Bucket Encryption
resource "aws_s3_bucket" "example" {
bucket = "example-bucket"
server_side_encryption_configuration {
rule {
apply_server_side_encryption_by_default {
sse_algorithm = "AES256"
}
}
}
}
This simple configuration ensures that all objects in your S3 bucket are encrypted by default using server-side encryption with AES-256.
Key Takeaways:
- Always encrypt data both at rest and in transit.
- Use AWS KMS for managing encryption keys securely.
4. Lock Down Your Network
In addition to managing who has access to your AWS resources, you also need to control which networks can access them. AWS’s Virtual Private Cloud (VPC), Security Groups, and Network Access Control Lists (NACLs) are crucial for this.
Example: Security Group to Allow Only HTTP/HTTPS Traffic
resource "aws_security_group" "web_sg" {
name = "web-sg"
description = "Allow HTTP and HTTPS inbound"
ingress {
from_port = 80
to_port = 80
protocol = "tcp"
cidr_blocks = ["0.0.0.0/0"]
}
ingress {
from_port = 443
to_port = 443
protocol = "tcp"
cidr_blocks = ["0.0.0.0/0"]
}
egress {
from_port = 0
to_port = 0
protocol = "-1"
cidr_blocks = ["0.0.0.0/0"]
}
}
This example creates a security group that only allows HTTP and HTTPS traffic while allowing all outgoing traffic.
Key Takeaways:
- Use Security Groups and NACLs to limit traffic to and from your resources.
- Put sensitive resources like databases in private subnets.
5. Automate Security Audits
AWS offers tools like AWS Config and CloudTrail to track changes and monitor resource configurations, helping you stay compliant with security best practices. Terraform can help you set up these services to automatically audit your infrastructure.
Example: Enabling AWS CloudTrail
resource "aws_cloudtrail" "example" {
name = "example-trail"
s3_bucket_name = aws_s3_bucket.example.bucket
is_multi_region_trail = true
}
With this setup, AWS CloudTrail will log every API call made in your AWS account, making it easy to audit and trace any changes.
Key Takeaways:
- Use AWS Config and CloudTrail to monitor and track resource changes.
- Automate compliance checks and security audits to avoid manual effort.
6. Be Ready for Incidents
Despite your best efforts, security incidents may still happen. Terraform can help you set up tools like AWS GuardDuty and Security Hub to monitor and detect potential threats in real-time.
Example: Provisioning AWS GuardDuty
resource "aws_guardduty_detector" "gd" {
enable = true
}
GuardDuty helps detect things like unusual API activity or unexpected behavior in your EC2 instances.
Key Takeaways:
- Use GuardDuty for continuous threat detection.
- Automate responses to common security threats where possible.
7. Enforce Multi-Factor Authentication (MFA)
For sensitive tasks, you should require Multi-Factor Authentication (MFA). Terraform can help enforce MFA for IAM users or roles to add an extra layer of protection.
Example: Requiring MFA for IAM Users
resource "aws_iam_user" "example" {
name = "user"
}
resource "aws_iam_user_policy" "require_mfa" {
name = "require_mfa"
user = aws_iam_user.example.name
policy = jsonencode({
"Version": "2012-10-17",
"Statement": [
{
"Effect": "Deny",
"Action": "*",
"Resource": "*",
"Condition": {
"BoolIfExists": {
"aws:MultiFactorAuthPresent": "false"
}
}
}
]
})
}
This policy denies any action unless the user is authenticated with MFA.
Key Takeaways:
- Enforce MFA for users with high-privilege roles.
- Regularly audit MFA use across your account.
Wrapping Up
Using Terraform to handle security on AWS not only ensures that your infrastructure is set up securely, but also gives you the ability to automate, repeat, and scale security best practices across your environment. By following these guidelines—limiting permissions, securing secrets, encrypting data, controlling network access, auditing configurations, monitoring threats, and enforcing MFA—you’ll be well on your way to building a secure, compliant AWS environment.