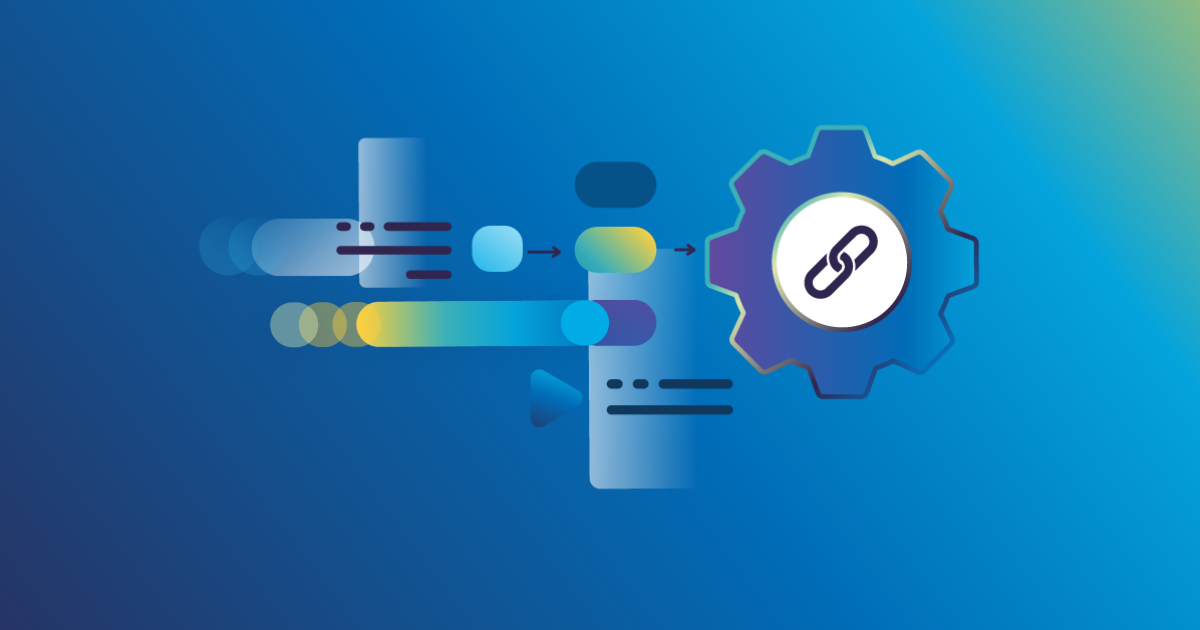
With the rise of language models like OpenAI's GPT-4, developing applications that harness the power of natural language processing (NLP) has become increasingly feasible and valuable for various domains. However, building robust language model (LM) applications involves more than just querying a model with text inputs; it requires chains, agents, memory, and often integrations with other tools to achieve complex tasks.
LangChain is a library designed specifically for this purpose, offering a set of tools that simplifies the creation of multi-step workflows and sophisticated language model applications.
Core Components of LangChain
LangChain has several core components that provide modularity and flexibility:
-
Chains: Chains are sequences of steps or components connected to perform a task. They allow for structured workflows where each step builds on the previous one.
-
Agents: Agents in LangChain are autonomous entities that can make decisions about which actions to take next based on the input they receive. Agents often decide which tools to use and how to use them.
-
Memory: Memory enables models to "remember" context across interactions, so they can refer to previous data during the workflow. This is useful for multi-turn conversations and applications where context over time is essential.
-
Tools: LangChain can be extended with tools like search engines, calculators, and APIs to provide additional functionalities beyond just text generation.
Getting Started with LangChain
To use LangChain, you’ll need to install it with pip:
pip install langchain
You’ll also need access to a language model provider like OpenAI or Hugging Face. For this example, we’ll assume you have an OpenAI API key.
Example 1: Building a Simple Chain
Let's start by creating a basic question-answering application using a Chain.
from langchain import OpenAI, LLMChain
from langchain.prompts import PromptTemplate
# Initialize the language model
llm = OpenAI(api_key="your_openai_api_key", model="gpt-4")
# Define the prompt template
prompt = PromptTemplate(
input_variables=["question"],
template="Answer the question concisely: {question}"
)
# Create a simple chain
qa_chain = LLMChain(llm=llm, prompt=prompt)
# Run the chain
question = "What is the capital of France?"
answer = qa_chain.run({"question": question})
print(answer) # Expected output: "Paris"
In this example, LLMChain
is used to create a simple question-answering chain. The PromptTemplate
formats the input question into a specific template that the model understands, and then LLMChain
passes it through the language model.
Example 2: Adding Memory for a Multi-Turn Conversation
Now, let’s extend this by adding memory, so the language model can remember context across multiple questions in a conversation.
from langchain import ConversationChain
from langchain.memory import ConversationBufferMemory
# Create a memory object
memory = ConversationBufferMemory()
# Define a conversational chain with memory
conversation = ConversationChain(
llm=llm,
memory=memory
)
# Start a conversation
print(conversation.run("Who won the FIFA World Cup in 2018?"))
print(conversation.run("Who was the runner-up?"))
# The model should remember the topic and provide contextually relevant responses
In this example, ConversationBufferMemory
stores the conversation context, allowing the model to respond with awareness of prior interactions.
Example 3: Using Agents for Tool Integration
Agents are particularly powerful because they can be configured to decide which tools to use depending on the user's input. Here, we will create an agent that uses a search engine and a calculator to answer more complex queries.
First, install the additional dependencies if needed:
pip install langchain[agents]
from langchain.agents import initialize_agent, Tool
from langchain.tools import Calculator, BingSearchAPI
# Initialize the tools
calc_tool = Calculator()
search_tool = BingSearchAPI(api_key="your_bing_api_key")
# Register tools with LangChain
tools = [
Tool(name="Calculator", func=calc_tool.run, description="Perform calculations"),
Tool(name="Search", func=search_tool.run, description="Search the web for answers")
]
# Create the agent
agent = initialize_agent(tools=tools, llm=llm, agent="zero-shot-react-description")
# Ask the agent a complex question
response = agent.run("What is the square root of 144? Also, who is the current president of the USA?")
print(response)
In this example, the agent determines which tool (calculator or search engine) to use based on the question. For instance, it will use the calculator for mathematical queries and the search tool for real-world information.
Example 4: Building a Summarization Pipeline
LangChain also simplifies building specialized applications like document summarization by combining its components.
from langchain import OpenAI, LLMChain
from langchain.prompts import PromptTemplate
# Define the summarization prompt
prompt = PromptTemplate(
input_variables=["text"],
template="Please summarize the following text: {text}"
)
# Initialize the chain for summarization
summarization_chain = LLMChain(llm=llm, prompt=prompt)
# Run the summarization
text = """LangChain is a library that simplifies building complex applications with language models. It provides modular components
such as chains, agents, memory, and tools that allow developers to create workflows involving multi-turn conversations,
decision-making agents, and even tool integration."""
summary = summarization_chain.run({"text": text})
print(summary)
Here, the chain applies a summarization prompt to the input text, generating a concise summary. Such a pipeline could be extended to summarize lengthy documents, reports, or articles automatically.
Example 5: Creating a Custom Chain with Multiple Steps
LangChain supports combining multiple chains to perform complex workflows. Here’s an example of a custom chain for summarizing and then analyzing text.
from langchain.chains import SequentialChain
from langchain.prompts import PromptTemplate
# Step 1: Summarize the text
summary_prompt = PromptTemplate(
input_variables=["text"],
template="Summarize the following content: {text}"
)
summarize_chain = LLMChain(llm=llm, prompt=summary_prompt)
# Step 2: Analyze the summary
analysis_prompt = PromptTemplate(
input_variables=["summary"],
template="Analyze the main points of the summary and provide key insights: {summary}"
)
analyze_chain = LLMChain(llm=llm, prompt=analysis_prompt)
# Combine the chains into a SequentialChain
summary_analysis_chain = SequentialChain(
chains=[summarize_chain, analyze_chain],
input_variables=["text"],
output_variables=["summary", "analysis"]
)
# Run the custom chain
text = "LangChain simplifies complex workflows with language models. It allows for memory, tool use, and agents to create robust applications."
output = summary_analysis_chain.run({"text": text})
print("Summary:", output["summary"])
print("Analysis:", output["analysis"])
This example demonstrates how multiple chains can be combined sequentially to perform a multi-step workflow, where the output from one step becomes the input for the next.
Conclusion
LangChain is a powerful and flexible library that extends the capabilities of language models by enabling complex workflows, multi-turn memory, tool usage, and agents. Its modular components allow developers to quickly experiment with and deploy NLP applications that go beyond single-response tasks, making it highly suited for applications in customer support, research assistance, content generation, and more.
LangChain makes it possible to build sophisticated applications with language models, opening up new avenues for NLP development. Whether you’re working on conversational agents, data summarization, or context-rich applications, LangChain provides the building blocks to bring these projects to life.