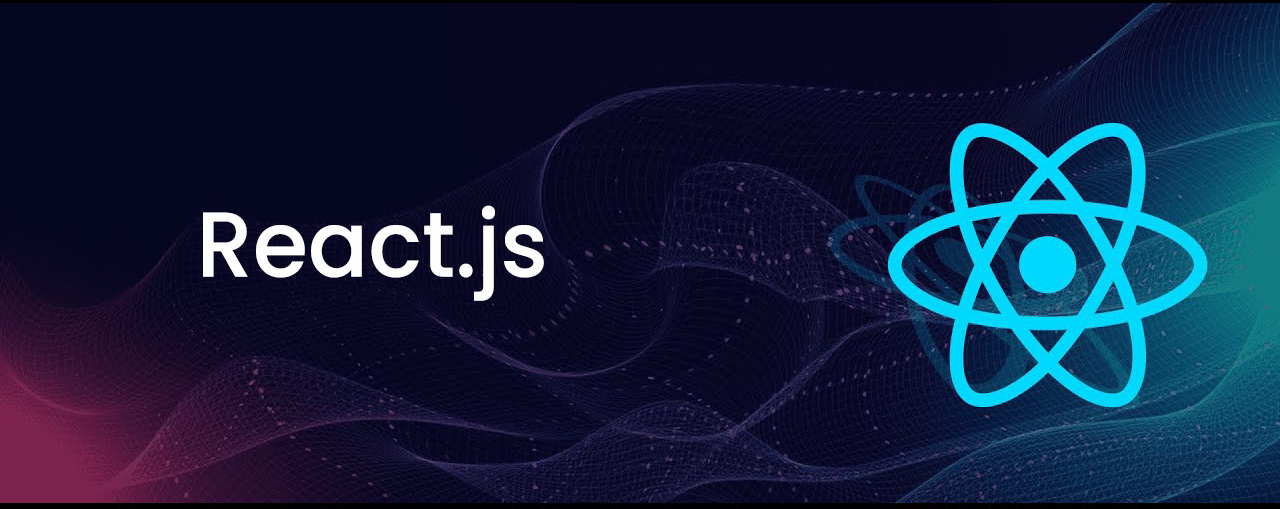
In the pursuit of crafting performant React applications, employing smart techniques like memoization can significantly enhance efficiency. Let's uncover how memoization optimizes rendering and boosts performance in React.
Understanding Memoization
Memoization is a technique that involves caching the results of expensive function calls and reusing them when the same computation occurs again. In React, the useMemo
hook serves as a powerful tool for implementing memoization.
Smart Trick: Optimize Rendering with useMemo
Consider a scenario where a component's render method contains computationally intensive calculations or complex data processing. By utilizing useMemo
, React intelligently memorizes the calculated value and only recomputes it when necessary, preventing unnecessary re-renders and enhancing performance.
Example: Memoizing Expensive Calculations
Let's illustrate this with an example. Suppose we have a component that performs a costly computation:
import React, { useMemo } from 'react';
const ExpensiveComponent = ({ data }) => {
const complexCalculation = useMemo(() => {
// Perform complex calculations based on data
// Example: Summing up an array of numbers
return data.reduce((acc, curr) => acc + curr, 0);
}, [data]); // Dependency array
return (
<div>
{/* Render the calculated value */}
<p>Result: {complexCalculation}</p>
</div>
);
};
export default ExpensiveComponent;
In this example, the useMemo
hook memoizes the result of the complexCalculation
based on the data
dependency. If the data
prop remains unchanged between renders, React reuses the cached result, avoiding unnecessary recalculations.
Benefits of Memoization in React
- Performance Optimization: Memoization prevents redundant calculations, leading to faster rendering and improved application performance, especially in components handling large datasets or complex logic.
- Preventing Unnecessary Renders: By memoizing values, React avoids unnecessary component renders, reducing the overall rendering workload and enhancing the user experience.
Considerations and Best Practices
- Identify Costly Operations: Apply memoization selectively to components or functions involving computationally expensive operations.
- Dependency Arrays: Ensure to define accurate dependency arrays in
useMemo
to trigger recalculation only when necessary.
Conclusion
Memoization in React, powered by useMemo
, emerges as a smart trick to optimize rendering performance by caching computed values intelligently. By strategically applying memoization to handle expensive computations, React developers can significantly enhance the efficiency and responsiveness of their applications.
In the pursuit of crafting high-performing React applications, leveraging memoization stands as a testament to the ingenuity of optimizing frontend performance.